java怎么调用微信接口(java怎么调用方法)
前言
微信是目前国内使用最广泛的社交平台之一,而我们的很多应用中需要与微信进行交互,获取一些数据或者处理一些业务。本文将分析Java如何调用微信接口,来帮助读者理解和使用微信API。
微信接口
微信提供了各种类型的API,包括用户信息、消息发送、支付等等。在Java中调用微信API的技巧与在其他编程语言中相似。我们需要一个http客户端来向微信API发送请求并解析json响应。不过,在使用微信API之前,我们需要申请一个公众号,并获取一个APPID和APPSECRET。这些值将用于调用微信API时进行身份验证。
示例
下面是一个Java程序,演示如何向微信API发送请求,以及如何获取用户信息。
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.net.URL; import java.nio.charset.StandardCharsets; import javax.net.ssl.HttpsURLConnection; import org.json.JSONException; import org.json.JSONObject; public class WeixinAPI { private static final String APPID = "your_appid"; private static final String APPSECRET = "your_appsecret"; public static void main(String[] args) throws IOException, JSONException { String accessToken = getAccessToken(); String openId = "your_openid"; JSONObject userInfo = getUserInfo(accessToken, openId); System.out.println(userInfo); } private static String getAccessToken() throws IOException, JSONException { String url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=" + APPID + "&secret=" + APPSECRET; JSONObject jsonObject = HttpsUtil.httpsRequest(url, "GET", null); return jsonObject.getString("access_token"); } private static JSONObject getUserInfo(String accessToken, String openId) throws IOException, JSONException { String url = "https://api.weixin.qq.com/cgi-bin/user/info?access_token=" + accessToken + "&openid=" + openId + "&lang=zh_CN"; JSONObject jsonObject = HttpsUtil.httpsRequest(url, "GET", null); return jsonObject; } private static class HttpsUtil { public static JSONObject httpsRequest(String requestUrl, String requestMethod, String outputStr) throws IOException, JSONException { URL url = new URL(requestUrl); HttpsURLConnection conn = (HttpsURLConnection) url.openConnection(); conn.setDoOutput(true); conn.setDoInput(true); conn.setUseCaches(false); conn.setRequestMethod(requestMethod); if ("GET".equalsIgnoreCase(requestMethod)) { conn.connect(); } if (outputStr != null) { conn.getOutputStream().write(outputStr.getBytes(StandardCharsets.UTF_8)); conn.getOutputStream().flush(); } InputStream inputStream = conn.getInputStream(); String jsonString = new BufferedReader(new InputStreamReader(inputStream, StandardCharsets.UTF_8)).lines().reduce("", (accumulator, actual) -> accumulator + actual); return new JSONObject(jsonString); } } }
在上面的代码中,我们调用getAccessToken方法获取访问令牌。这个方法发送一个GET请求到微信API,获取一个包含访问令牌的json响应。我们将访问令牌存储在accessToken变量中。
接下来,我们调用getUserInfo方法来获取指定用户的详细信息。这个方法发送GET请求到微信API,获取一个包含用户信息的json响应。我们将用户信息存储在JSONObject变量userInfo中,打印输出。
运行这个Java程序,你也可以获得自己的微信用户信息。这个程序只是一个起点,你可以进一步探索微信API,更好地理解它们的功能和用法。
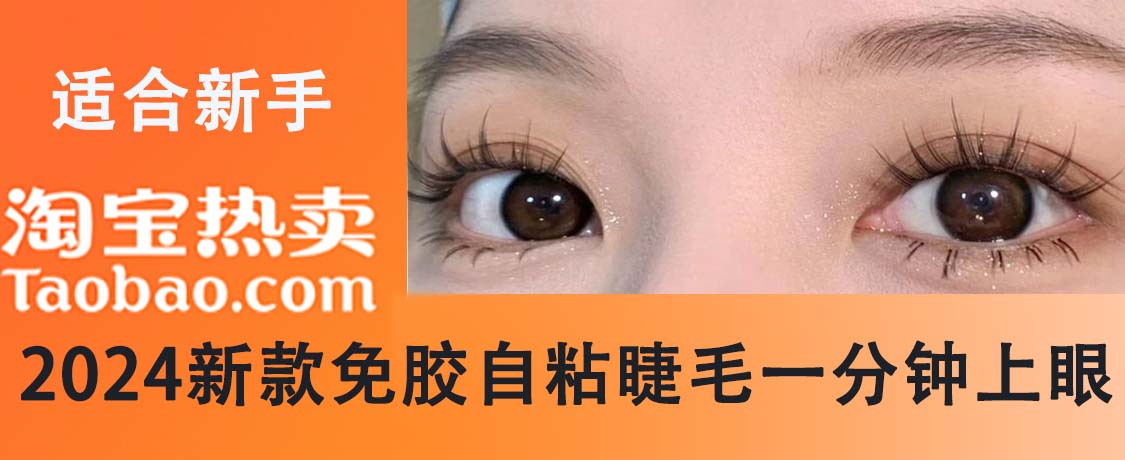
声明:本站所有文章资源内容,如无特殊说明或标注,均为采集网络资源。如若本站内容侵犯了原著者的合法权益,可联系本站删除。