js怎么获取当前时间(js获取当前时间年月日时分秒)
如何获取当前时间?
第一段落:js获取当前时间的方法
JavaScript中获取当前时间的方法很简单。可以使用JavaScript中内置的Date对象,调用它的方法getHours()、getMinutes()和getSeconds()来获取当前时间的时、分、秒。代码如下:
var date = new Date();
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
这段代码可以获取当前时间的时、分、秒并存储在变量hours、minutes和seconds中。
第二段落:格式化当前时间
如果需要按照一定的格式显示当前时间,可以使用以下方法。可以先获取当前时间的年、月、日、时、分、秒,然后使用字符串拼接的方式按照需要的格式显示时间。代码如下:
var date = new Date();
var year = date.getFullYear();
var month = date.getMonth() + 1;
var day = date.getDate();
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
var strDate = year + "-" + month + "-" + day + " " + hours + ":" + minutes + ":" + seconds;
这段代码可以将当前时间按照“年-月-日 时:分:秒”的格式显示,并存储在变量strDate中。
第三段落:自定义当前时间格式
如果需要按照自定义的时间格式显示当前时间,可以使用JavaScript中的正则表达式来处理,并使用字符串的replace()方法进行替换。代码如下:
var date = new Date();
var year = date.getFullYear();
var month = date.getMonth() + 1;
var day = date.getDate();
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
var format = "YYYY/MM/DD hh:mm:ss";
var strDate = format.replace(/YYYY/g, year)
.replace(/MM/g, month)
.replace(/DD/g, day)
.replace(/hh/g, hours)
.replace(/mm/g, minutes)
.replace(/ss/g, seconds);
这段代码可以根据自定义的格式显示当前时间,并存储在变量strDate中。
总结
以上就是JavaScript中获取当前时间的方法,可以使用Date对象的getHours()、getMinutes()和getSeconds()方法来获取当前时间的时、分、秒,按照固定的格式或自定义的格式显示当前时间。
JavaScript中的日期格式化还有许多其他的方法,比如使用moment.js这个库。根据需要选择适合自己的方法即可。
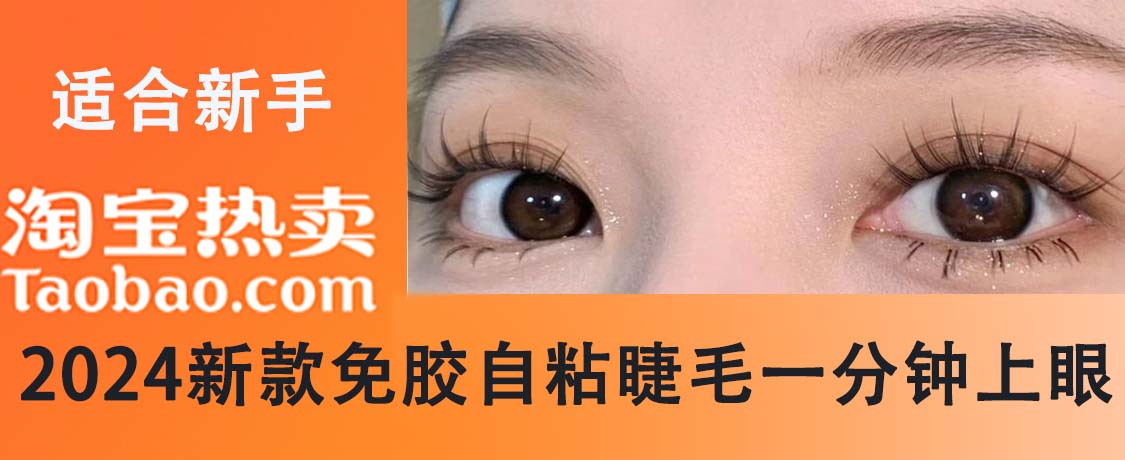
声明:本站所有文章资源内容,如无特殊说明或标注,均为采集网络资源。如若本站内容侵犯了原著者的合法权益,可联系本站删除。